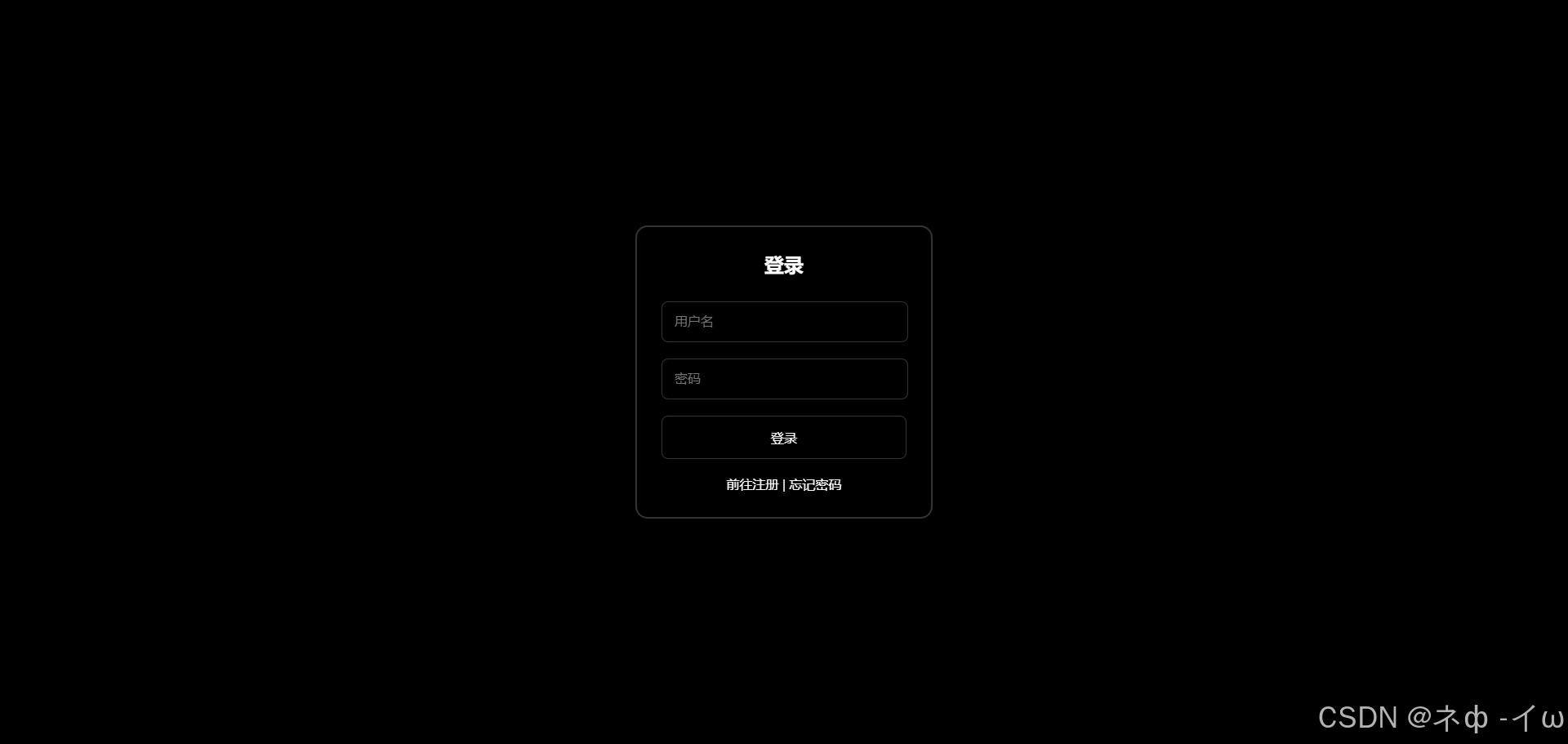
html">
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>登录</title>
<style>css">
body {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
background-color: #000;
font-family: Arial, sans-serif;
user-select: none;
}
body::before {
content: "";
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
background: inherit;
filter: blur(10px);
z-index: -1;
}
.login-box {
background-color: rgba(0, 0, 0, 0.5);
padding: 30px;
border-radius: 15px;
backdrop-filter: blur(10px);
width: 300px;
border: 2px solid rgba(255, 255, 255, 0.2);
transition: all 0.3s ease;
position: relative;
}
.login-box h2 {
margin: 0 0 30px 0;
color: #fff;
text-align: center;
}
.login-box input {
width: calc(100% - 30px);
padding: 15px;
margin-bottom: 20px;
border: 1px solid rgba(255, 255, 255, 0.2);
border-radius: 8px;
font-size: 16px;
outline: none;
transition: all 0.3s ease;
color: #fff;
background-color: rgba(0, 0, 0, 0.3);
-webkit-appearance: none;
-moz-appearance: none;
appearance: none;
-webkit-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
.login-box input:focus {
background-color: rgba(0, 0, 0, 0.5);
border-color: rgba(255, 255, 255, 0.4);
}
.login-box button {
width: 100%;
padding: 15px;
border: 1px solid rgba(255, 255, 255, 0.2);
border-radius: 8px;
background-color: rgba(0, 0, 0, 0.5);
cursor: pointer;
font-size: 16px;
color: #fff;
transition: all 0.3s ease;
}
.login-box button:hover {
background-color: rgba(0, 0, 0, 0.7);
border-color: rgba(255, 250, 255, 0.4);
}
.message {
color: #fff;
text-align: center;
padding: 10px;
border-radius: 8px;
overflow: hidden;
position: absolute;
left: 0;
right: 0;
bottom: -60px;
transition: opacity 0.3s ease;
opacity: 0;
margin: 0 auto;
background-color: rgba(0, 0, 255, 0.3);
}
.link {
color: #fff;
text-align: center;
margin-top: 20px;
cursor: pointer;
}
</style>
</head>
<body>
<div class="login-box">
<h2>登录</h2>
<form action="#">
<div class="input-container">
<input type="text" id="username" placeholder="用户名" autocomplete="off">
</div>
<div class="input-container">
<input type="password" id="password" placeholder="密码" autocomplete="off">
</div>
<button type="submit" id="loginButton">登录</button>
</form>
<div class="message" id="message"></div>
<div class="link">
<span id="registerLink">前往注册</span> |
<span id="forgetPasswordLink">忘记密码</span>
</div>
</div>
<script>
document.getElementById('loginButton').addEventListener('click', function(event) {
event.preventDefault();
const username = document.getElementById('username').value;
const password = document.getElementById('password').value;
const message = document.getElementById('message');
if (username && password) {
message.innerHTML = '登录成功';
showMessage();
clearInputsAndMessage();
setTimeout(() => {
window.location.href = 'index.html';
}, 300);
} else {
message.innerHTML = '登录失败,请输入用户名和密码';
showMessage();
clearInputsAndMessage();
}
});
document.getElementById('registerLink').addEventListener('click', function() {
window.location.href = 'register.html';
});
document.getElementById('forgetPasswordLink').addEventListener('click', function() {
window.location.href = 'resetPassword.html';
});
function showMessage() {
const message = document.getElementById('message');
message.style.opacity = '1';
}
function clearInputsAndMessage() {
const message = document.getElementById('message');
const username = document.getElementById('username');
const password = document.getElementById('password');
setTimeout(() => {
message.innerHTML = '';
message.style.opacity = '0';
username.value = '';
password.value = '';
}, 3000);
}
</script>
</body>
</html>
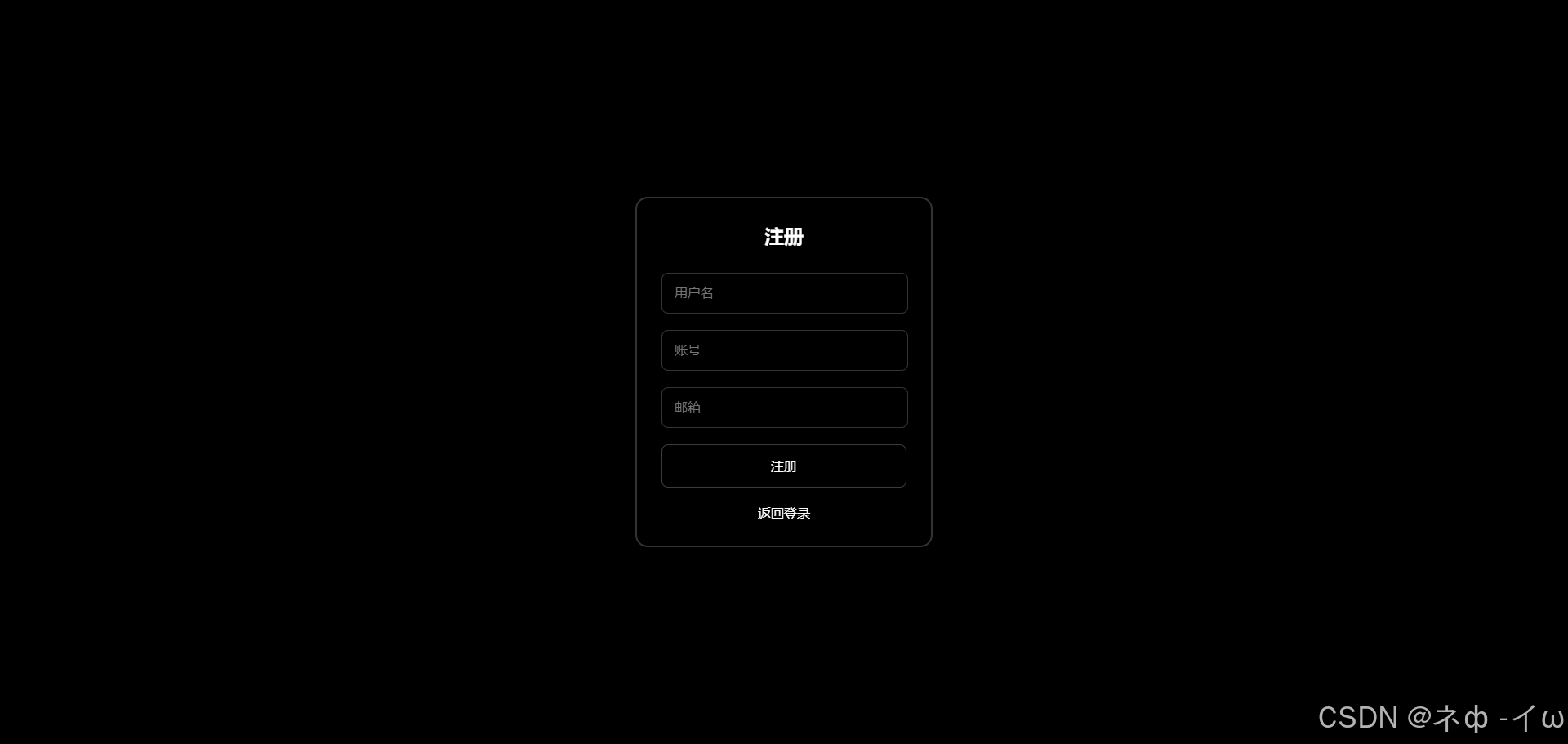
html">
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>注册</title>
<style>css">
body {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
background-color: #000;
font-family: Arial, sans-serif;
user-select: none;
}
body::before {
content: "";
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
background: inherit;
filter: blur(10px);
z-index: -1;
}
.register-box {
background-color: rgba(0, 0, 0, 0.5);
padding: 30px;
border-radius: 15px;
backdrop-filter: blur(10px);
width: 300px;
border: 2px solid rgba(255, 255, 255, 0.2);
transition: all 0.3s ease;
position: relative;
}
.register-box h2 {
margin: 0 0 30px 0;
color: #fff;
text-align: center;
}
.register-box input {
width: calc(100% - 30px);
padding: 15px;
margin-bottom: 20px;
border: 1px solid rgba(255, 255, 255, 0.2);
border-radius: 8px;
font-size: 16px;
outline: none;
transition: all 0.3s ease;
color: #fff;
background-color: rgba(0, 0, 0, 0.3);
-webkit-appearance: none;
-moz-appearance: none;
appearance: none;
-webkit-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
.register-box input:focus {
background-color: rgba(0, 0, 0, 0.5);
border-color: rgba(255, 255, 255, 0.4);
}
.register-box button {
width: 100%;
padding: 15px;
border: 1px solid rgba(255, 255, 255, 0.2);
border-radius: 8px;
background-color: rgba(0, 0, 0, 0.5);
cursor: pointer;
font-size: 16px;
color: #fff;
transition: all 0.3s ease;
}
.register-box button:hover {
background-color: rgba(0, 0, 0, 0.7);
border-color: rgba(255, 250, 255, 0.4);
}
.message {
color: #fff;
text-align: center;
padding: 10px;
border-radius: 8px;
overflow: hidden;
position: absolute;
left: 0;
right: 0;
bottom: -60px;
transition: opacity 0.3s ease;
opacity: 0;
margin: 0 auto;
background-color: rgba(0, 0, 255, 0.3);
}
.link {
color: #fff;
text-align: center;
margin-top: 20px;
cursor: pointer;
}
</style>
</head>
<body>
<div class="register-box">
<h2>注册</h2>
<form action="#">
<div class="input-container">
<input type="text" id="username" placeholder="用户名" autocomplete="off">
</div>
<div class="input-container">
<input type="text" id="account" placeholder="账号" autocomplete="off">
</div>
<div class="input-container">
<input type="email" id="email" placeholder="邮箱" autocomplete="off">
</div>
<button type="submit" id="registerButton">注册</button>
</form>
<div class="message" id="message"></div>
<div class="link">
<span id="loginLink">返回登录</span>
</div>
</div>
<script>
document.getElementById('registerButton').addEventListener('click', function(event) {
event.preventDefault();
const username = document.getElementById('username').value;
const account = document.getElementById('account').value;
const email = document.getElementById('email').value;
const message = document.getElementById('message');
if (username && account && email) {
message.innerHTML = '注册成功';
showMessage();
clearInputsAndMessage();
} else {
message.innerHTML = '注册失败,请输入用户名、账号和邮箱';
showMessage();
clearInputsAndMessage();
}
});
document.getElementById('loginLink').addEventListener('click', function() {
window.location.href = 'login.html';
});
function showMessage() {
const message = document.getElementById('message');
message.style.opacity = '1';
}
function clearInputsAndMessage() {
const message = document.getElementById('message');
const username = document.getElementById('username');
const account = document.getElementById('account');
const email = document.getElementById('email');
setTimeout(() => {
message.innerHTML = '';
message.style.opacity = '0';
username.value = '';
account.value = '';
email.value = '';
}, 3000);
}
</script>
</body>
</html>
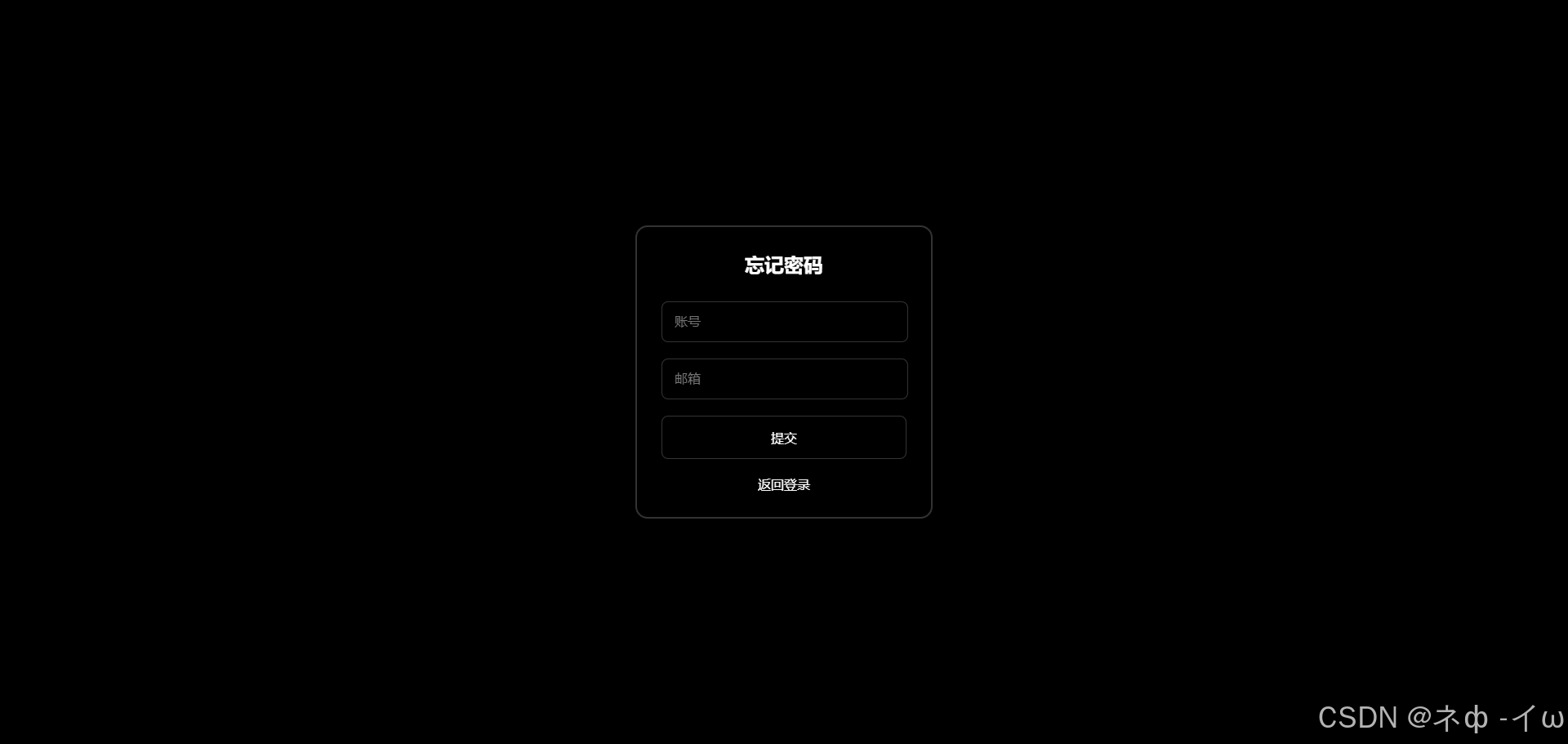
html">
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>忘记密码页面</title>
<style>css">
body {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
background-color: #000;
font-family: Arial, sans-serif;
user-select: none;
}
body::before {
content: "";
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
background: inherit;
filter: blur(10px);
z-index: -1;
}
.reset-box {
background-color: rgba(0, 0, 0, 0.5);
padding: 30px;
border-radius: 15px;
backdrop-filter: blur(10px);
width: 300px;
border: 2px solid rgba(255, 255, 255, 0.2);
transition: all 0.3s ease;
position: relative;
}
.reset-box h2 {
margin: 0 0 30px 0;
color: #fff;
text-align: center;
}
.reset-box input {
width: calc(100% - 30px);
padding: 15px;
margin-bottom: 20px;
border: 1px solid rgba(255, 255, 255, 0.2);
border-radius: 8px;
font-size: 16px;
outline: none;
transition: all 0.3s ease;
color: #fff;
background-color: rgba(0, 0, 0, 0.3);
-webkit-appearance: none;
-moz-appearance: none;
appearance: none;
-webkit-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
.reset-box input:focus {
background-color: rgba(0, 0, 0, 0.5);
border-color: rgba(255, 255, 255, 0.4);
}
.reset-box button {
width: 100%;
padding: 15px;
border: 1px solid rgba(255, 255, 255, 0.2);
border-radius: 8px;
background-color: rgba(0, 0, 0, 0.5);
cursor: pointer;
font-size: 16px;
color: #fff;
transition: all 0.3s ease;
}
.reset-box button:hover {
background-color: rgba(0, 0, 0, 0.7);
border-color: rgba(255, 250, 255, 0.4);
}
.message {
color: #fff;
text-align: center;
padding: 10px;
border-radius: 8px;
overflow: hidden;
position: absolute;
left: 0;
right: 0;
bottom: -60px;
transition: opacity 0.3s ease;
opacity: 0;
margin: 0 auto;
background-color: rgba(0, 0, 255, 0.3);
}
.link {
color: #fff;
text-align: center;
margin-top: 20px;
cursor: pointer;
}
</style>
</head>
<body>
<div class="reset-box">
<h2>忘记密码</h2>
<form action="#">
<div class="input-container">
<input type="text" id="account" placeholder="账号" autocomplete="off">
</div>
<div class="input-container">
<input type="email" id="email" placeholder="邮箱" autocomplete="off">
</div>
<button type="submit" id="resetButton">提交</button>
</form>
<div class="message" id="message"></div>
<div class="link">
<span id="loginLink">返回登录</span>
</div>
</div>
<script>
document.getElementById('resetButton').addEventListener('click', function(event) {
event.preventDefault();
const account = document.getElementById('account').value;
const email = document.getElementById('email').value;
const message = document.getElementById('message');
if (account && email) {
message.innerHTML = '密码重置请求已提交,请查收邮件';
showMessage();
clearInputsAndMessage();
} else {
message.innerHTML = '请输入账号和邮箱';
showMessage();
clearInputsAndMessage();
}
});
document.getElementById('loginLink').addEventListener('click', function() {
window.location.href = 'login.html';
});
function showMessage() {
const message = document.getElementById('message');
message.style.opacity = '1';
}
function clearInputsAndMessage() {
const message = document.getElementById('message');
const account = document.getElementById('account');
const email = document.getElementById('email');
setTimeout(() => {
message.innerHTML = '';
message.style.opacity = '0';
account.value = '';
email.value = '';
}, 3000);
}
</script>
</body>
</html>
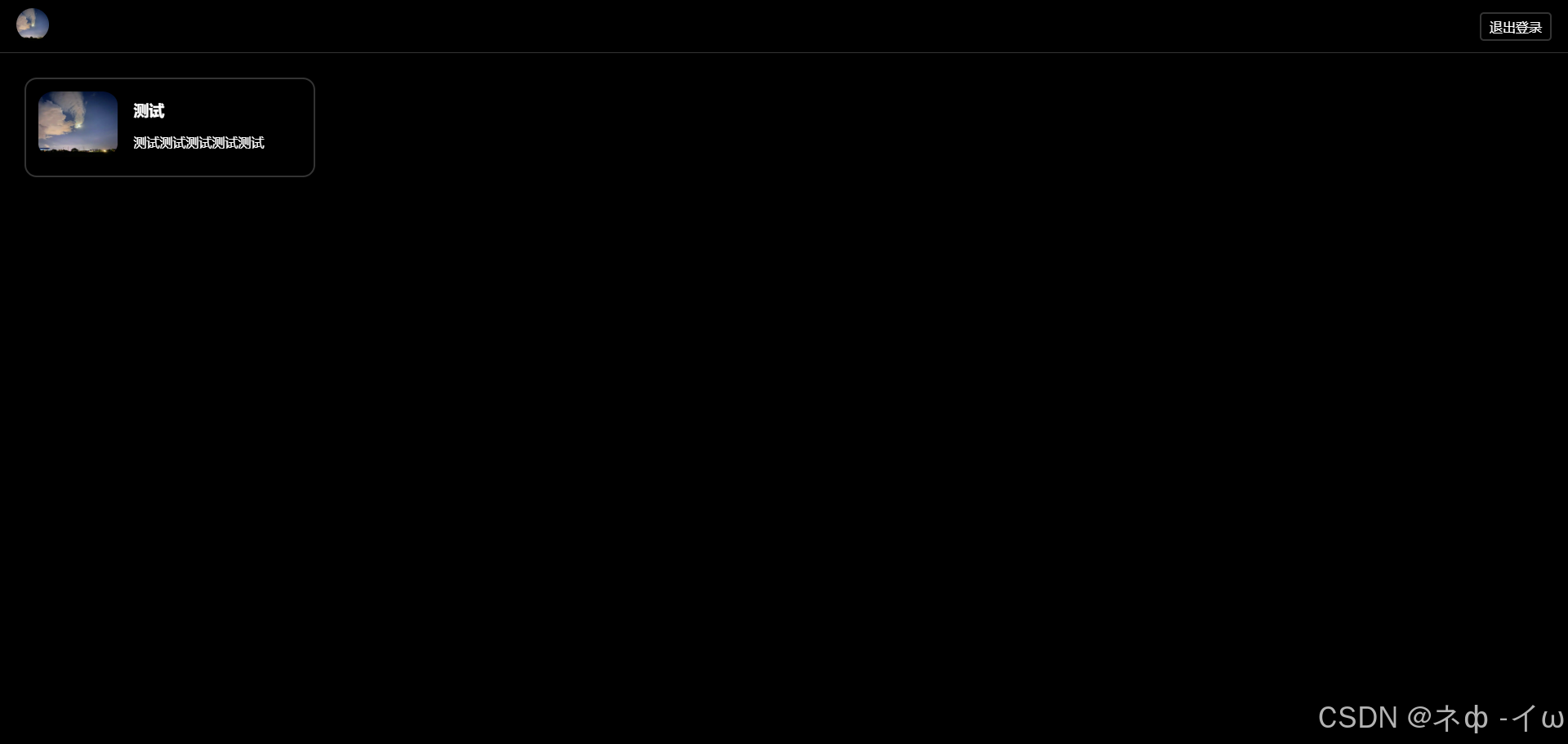
html">
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>首页</title>
<style>css">
body {
margin: 0;
padding: 0;
font-family: Arial, sans-serif;
background-color: #000;
user-select: none;
}
body::before {
content: "";
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
background: inherit;
filter: blur(10px);
z-index: -1;
}
.header {
display: flex;
justify-content: space-between;
align-items: center;
padding: 10px 20px;
background-color: rgba(0, 0, 0, 0.7);
border-bottom: 1px solid rgba(255, 255, 255, 0.2);
}
.system-logo {
height: 40px;
width: 40px;
border-radius: 50%;
}
.logout {
color: #fff;
cursor: pointer;
padding: 5px 10px;
border: 2px solid rgba(255, 255, 255, 0.2);
border-radius: 5px;
transition: background-color 0.3s ease;
}
.logout:hover {
background-color: rgba(255, 255, 255, 0.2);
}
.card-container {
display: flex;
flex-wrap: wrap;
justify-content: flex-start;
padding: 20px;
}
.card {
flex: 0 0 calc(20% - 20px);
background-color: rgba(0, 0, 0, 0.5);
border-radius: 15px;
backdrop-filter: blur(10px);
border: 2px solid rgba(255, 255, 255, 0.2);
margin: 10px;
padding: 15px;
box-sizing: border-box;
display: flex;
}
.logo {
flex: 0 0 30%;
height: 80px;
width: 80px;
margin-right: 10px;
border-radius: 20%;
user-drag: none;
}
.card-content {
flex: 0 0 70%;
padding-left: 10px;
}
.card-content h3 {
color: #fff;
margin: 10px 0;
}
.card-content p {
color: #fff;
}
.modal {
display: none;
position: fixed;
z-index: 1;
left: 50%;
top: 50%;
transform: translate(-50%, -50%);
width: 300px;
height: 150px;
overflow: auto;
background-color: rgba(0, 0, 0, 0.8);
border: 2px solid rgba(255, 255, 255, 0.2);
border-radius: 10px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.2);
}
.modal-content {
padding: 20px;
}
.modal-content p {
color: #fff;
text-align: center;
}
.modal-buttons {
display: flex;
justify-content: center;
margin-top: 20px;
}
.modal-buttons button {
margin: 0 10px;
padding: 10px 20px;
cursor: pointer;
border: none;
border-radius: 5px;
background-color: #007BFF;
color: #fff;
transition: background-color 0.3s ease;
}
.modal-buttons button:hover {
background-color: #0056b3;
}
</style>
</head>
<body>
<div class="header">
<div class="logo-container">
<img class="system-logo" src="https://img2.baidu.com/it/u=3565369971,2082314928&fm=253&app=138&size=w931&n=0&f=JPEG&fmt=auto?sec=1736960400&t=2bbb8a8e0be6e8ae7d1b4713751f1b0f" alt="System Logo" draggable="false">
</div>
<div class="logout" id="logout">退出登录</div>
</div>
<div class="card-container">
<div class="card">
<img class="logo" src="https://img2.baidu.com/it/u=3565369971,2082314928&fm=253&app=138&size=w931&n=0&f=JPEG&fmt=auto?sec=1736960400&t=2bbb8a8e0be6e8ae7d1b4713751f1b0f" alt="Card Logo" draggable="false">
<div class="card-content">
<h3>测试</h3>
<p>测试测试测试测试测试</p>
</div>
</div>
</div>
<div class="modal" id="modal">
<div class="modal-content">
<p>是否确认退出登录?</p>
<div class="modal-buttons">
<button id="confirmLogout">确认</button>
<button id="cancelLogout">取消</button>
</div>
</div>
</div>
<script>
document.getElementById('logout').addEventListener('click', function() {
document.getElementById('modal').style.display = 'block';
});
document.getElementById('confirmLogout').addEventListener('click', function() {
window.location.href = 'login.html';
document.getElementById('modal').style.display = 'none';
});
document.getElementById('cancelLogout').addEventListener('click', function() {
document.getElementById('modal').style.display = 'none';
});
</script>
</body>
</html>